Onsite Toolbar Tutorial
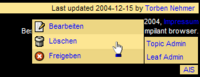
With MidCOM 2.0.0 components have the ability to add more detailed AIS link information to their NAP data as before. This allows for a quite efficient on-site maitainance without having to "walk through" the navigation tree both on-site and in AIS.
If you click on the image on the right, you will see what this litte tutorial will produce. Internet Explorer users beware, this is CSS 2.0 level code not supported by your standard-ignorant browser. Use the free and way more powerful alternative Mozilla instead. Also note, that the example I use here on this site is fixed to the German language, which is a decision of me, not a limitation of MidCOM.
The main goal for me at this point was to have all AIS entry points available quickly and easily, and with Mozilla only, as this is the premiere browser I use. The AIS rectangle at the bottom right of the page contains all information, and in case I don't have a mozilla ready, it links to the current edit URL as set by NAP. The leaf and topic editing popups is then added as a submenu if and only if the corresponding toolbars are non-empty.
The trick was a combination of the right CSS layout taken from the currently available CSS popup sampls available throughout the net.
Note, that this code does not assume the availability of MidCOM template. The standard menu is shown every time, using the anonymous user that is working with the site; this is due to the fact, that I'm using the classic Midgard HTTP basic auth in favor of MidCOM Template. Personal taste, that's all. Where neccessary, I'll give a short drop-in what to change if you are using MidCOM Template.
The MidCOM side
This part of the equation is the actually interesting part for those of you, who want to add a similar piece of code in general, with their own styling. It is all located within my page-style, of course. Lets take a look at actual code:
9: <?php
10: $aisbase = "http://www.nathan-syntronics.de/admin/";
11: $nap = new midcom_helper_nav();
12: $topic_id = $nap->get_current_node();
13: $leaf_id = $nap->get_current_leaf();
14: $fallback_url = $nap->edit_current_page_url($aisbase);
15: ?>
16: <UL ID="aispopup">
17: <LI>
18: <a href="&(fallback_url);">AIS</a>
19: <UL>
20: <LI>
21: <a href="&(aisbase);&(topic_id);/data/">Topic Admin</a>
22: <?php
23: $toolbar = $nap->get_node_toolbar($aisbase);
24: $toolbar->class_style = null;
25: echo $toolbar->render();
26: ?>
27: </li>
28: <?php
29: if ($leaf_id !== false)
30: {
31: $leaf_url = $nap->edit_current_page_url($aisbase);
32: ?>
33: <LI>
34: <a href="&(leaf_url);">Leaf Admin</a>
35: <?php
36: $toolbar = $nap->get_leaf_toolbar($aisbase);
37: $toolbar->class_style = null;
38: echo $toolbar->render();
39: ?>
40: </li>
41: <?php } ?>
42: </ul>
43: </li>
44: </UL>
As you can see, it is not very difficult. I do a bit of the NAP work myself so that I can distinguish between leaves and nodes at all times.
For both leaves and nodes I retrieve the toolbar class from the local NAP instance and render it directly. Since the main aispopup CSS rules will take care of all formatting, I clear the default CSS class applied to the toolbar in its constructor. Remember, that the toolbars will render themselves only, if at least one item is present. If not, the render-Call will give you an empty string and hence there will be no popup.
If you adapt this to your own site, you have to adapt line 7 above to the correct root URL of your AIS installation.
Both get_..._toolbar calls do not need an leaf or node ID as the default behavoir of these calls is to retrieve the toolbar for the currently active object.
For the sake of completness: The type sensitive check in line 29 is required, as leaves can have an id of 0 (which would evaluate to false).
As you can see, nothing special here. Hit your browsers View Source button to see the result at the bottom of the page.
MidCOM Template tweaks and Authentication notes
The code shown above will work as-is with MidCOM Template. But since the on-site code of MidCOM Template is authentication aware, you can hide the UL if no user is authenticated, showing a login link instead. Additionally, when logged in, you could add the name of the authenticated user and a logout link to the menu.
Important Note: The 2.0 release NAP toolbar code will not show you any toolbar items if you are not authenticated and either a topic owner or an administrator. If you, like me, prefer HTTP basic auth at your AIS, you need to patch the /lib/midcom/helper/nav.php file a bit using the patch nap-toolbar-noauth.patch, which you can find in the download section of this page.
CSS magic
As I have told in the introduction, the whole popup is solely done in CSS, with absolutely no JavaScript in it. Which is, why IE 6 does not know what to do with it.
The basic idea is taken from the Pure CSS menu demo of Eric Meyer, which bases the effect on this:
li ul {display: none;}
li:hover > ul {display: block;}
Of course, the actual code is a bit more complex, requiring a bit of absolute positioning to get the popup into the place where you want it. This is the complete CSS rules I have in use:
#someotherelement {
/* This is the element that has the #aispopup in it.
* it is important to add the statement below to it
* or else the relative positioning I do here to get
* the element to the bottom right of the page would
* not work.
*/
position: relative;
}
#aispopup {
display: block !important;
display: none;
position: absolute;
width: 2em;
right: 0px;
white-space: nowrap;
margin: 3px 0 0 0;
padding: 0;
text-align: center;
background-color: #edd683;
border: 1px solid black;
}
#aispopup li {
list-style-type: none;
position: relative;
margin: 0;
padding: 0;
font-size: small;
}
#aispopup li a {
display: block;
padding: 3px;
text-decoration: none;
}
#aispopup ul {
display: none;
margin: 0;
padding: 0;
}
#aispopup li a:hover {
background: #FDE693;
}
#aispopup > li {
margin: 0;
padding: 0;
}
#aispopup > li > a {
margin: 0;
padding: 0;
}
/* Generic Submenu shown right to the parent menu */
#aispopup li:hover > ul {
display: block;
position: absolute;
bottom: -1px;
right: 6em;
background-color: #edd683;
width: 12.5em;
text-align: left;
border: 1px solid black;
}
/* First-Level Submenu shown above the main menu, */
/* inherits all the default submenu settings */
#aispopup > li:hover > ul {
bottom: 1.1em;
right: -1px;
width: 6em;
}
/* Align the toolbar images correctly */
#aispopup li img {
vertical-align: top;
padding: 0 0.25em 0 0;
border: none;
}
Explaining this all in detail would go beyond the scope of this article, so I'll focus on some nifty details:
As you can see at the beginning of the list, you have to "activate" the absolute positioning in the element enclosing the AIS menu popup. You will find similar lines throughout the CSS code for this. The CSS-Discuss Wiki page AbsoluteLayouts covers this topic in more depth.
The main problem I have not yet been able to solve (please tell me, if you know a solution!), is the neccessity of setting the popup menus to a fixed width. I have not been able solve this issue yet, so you possibly need to tweak the widths given in the rules above a bit to match your site. I hope though, that my usage of em instead of px as a measurement unit keeps this at a minimum. It is especially important for the Generic Submenu rule, which needs to be adapted so that the broadest menu item fits into your menu. If you don't use n.n.orders or one of the similarily complex components of the net.nemein domain, you should be fine with the value above though.
You might also have noticed, that there are several places where I move a popup by -1px away from the original position. This is to account for the 1px border of the unordered lists in place. Actually, I have no idea if this is a bug in Mozilla or a correct interpretation of CSS.
On a final note, I have decided to hide the AIS menu in IE completly, using the !important-Hack, it is unusable there anyway, and even the basic formatting is ignored by IE which would result in a weird looking page.
Further pointers around this topic
- The CSS-Discuss Wiki is in general an excellent resource about all types of CSS questions.
- They too have a collection about List-Menus.
- A big thank goes to Eric Mayer, I have taken most code from his Pure CSS Menu demo.
- If you want to make this menu work under IE6 you might take a look at the Suckerfish Dropdown code, though their multi-level css style rule code seems a bit inefficient compoared to the one of Eric Mayer. I have no idea if it is really neccessary though.
Downloads
File | Size | Last modified |
---|---|---|
MidCOM NAP toolbar no-auth patch, against 2.0.0 nav.php. | 297 Byte | 2004-12-20 |